SMSGPT - Your Phone as SMS Gateway
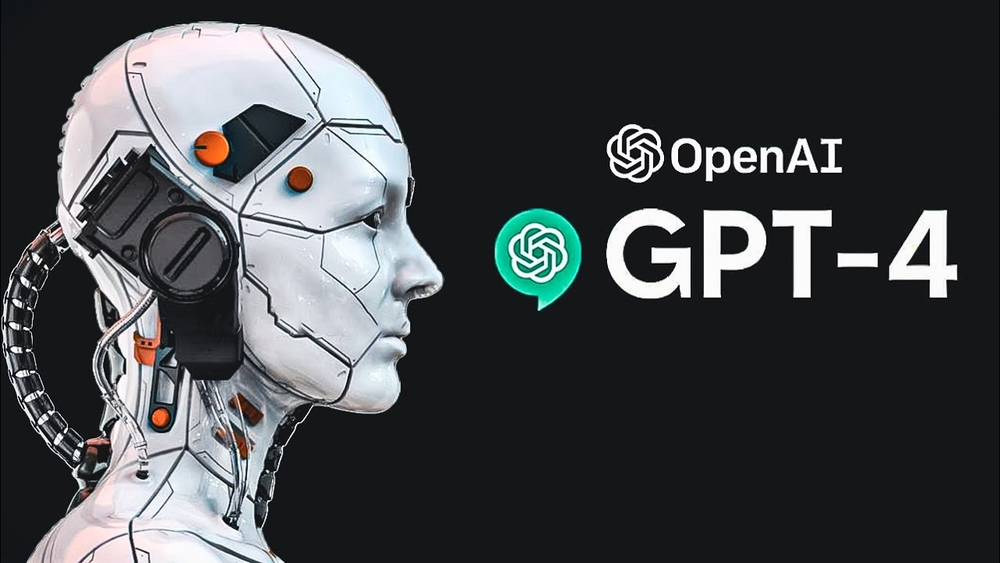
Are you curious about the endless possibilities of integrating ChatGPT into SMS? Do you want to explore the exciting world of artificial intelligence and messaging? Well, get ready to experience the cutting-edge technology of ChatGPT and SMS integration. By bringing ChatGPT to your SMS service, you can unleash the power of natural language processing and machine learning, creating intelligent and dynamic conversations like never before. And with the use of your Android phone as an SMS gateway through the Androidhelper library.
Get ready to embark on a journey of discovery and unlock the true potential of ChatGPT and SMS integration. Let's dive in and explore this exciting and innovative technology together!
To integrate ChatGPT with your SMS service using Python, you will need to have Python installed on your system, access to ChatGPT, and the required Python libraries, including requests, Flask, and OpenAI.
To use your Android phone as an SMS gateway, you'll need to install the QPython3L and Androidhelper library and configure your phone as a SMS Gateway. By meeting these requirements, you can seamlessly integrate ChatGPT with your SMS service and unlock the full potential of artificial intelligence and messaging.
On your Machine, you'll need to install two libraries by doing.
pip install openai
pip install Flask
On your phone, you'll need to install QPython3L. This is exactly the release qpython_3s_2020-01-25_google.apk
Open the console and install the requests library
pip3 install requests==2.17.1
Congratulations on meeting all the requirements to integrate ChatGPT with your SMS service using Python! You are now one step closer to unlocking the true potential of artificial intelligence and messaging.
By combining the power of ChatGPT with the convenience of SMS communication, you can now create dynamic and intelligent conversations that will revolutionize the way you interact with people. Get ready to embark on an exciting journey of innovation and explore the endless possibilities that await you. The future of messaging is here, and you're at the forefront of this exciting new technology. So, let's get started and bring ChatGPT to your SMS service!
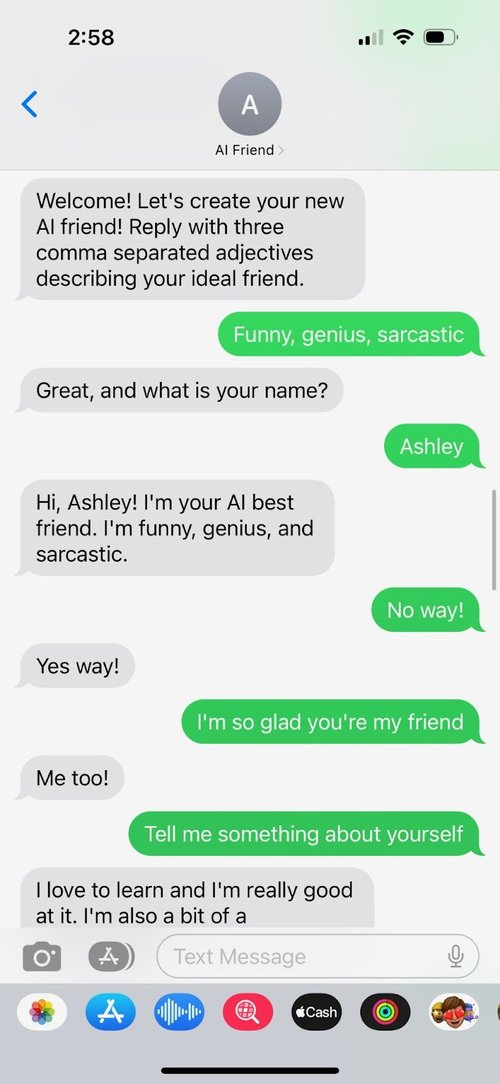
THE FLOW OF THIS PROJECT
Integrating ChatGPT into SMS service with the help of Android and QPython can open up a world of possibilities for dynamic and intelligent messaging. With the Androidhelper library, you can easily fetch the content of incoming SMS messages and the sender's phone number on your Android phone, which can then be sent to ChatGPT API to receive insightful and interactive responses. These responses can then be relayed back to the sender's phone number using Android helper library functions, allowing for a seamless messaging experience that's both engaging and informative.
By following these steps, you can bring the power of artificial intelligence to your SMS communication with ease, while also streamlining your messaging process. With the potential to personalize responses, improve engagement and interaction, and save time and effort, the integration of ChatGPT with SMS services can have a profound impact on the way you communicate with your audience. Get ready to explore the possibilities of AI and messaging and unlock the full potential of your SMS service with ChatGPT!
CHAT GPT WRAPPER
'''
File: gpt35.py
Purpose: Wrapping OpenAI ChatGPT API calls
For: SMSGPT
Author: Mohamed
License: GNU General Public License version 2.0 or Later version
'''
import typing
import openai
import json
openai.api_key = 'PUT-YOUR-API-KEY-HERE'
def chatgpt35(msg: str) -> any:
completion = openai.ChatCompletion.create(
model="gpt-3.5-turbo",
messages=[
{"role": "user", "content": msg}
]
)
return json.loads(json.dumps(completion.choices[0].message))['content']
The code above defines a function called chatgpt35 that takes a string parameter msg and returns an unspecified type of value. Within the function, the code utilizes OpenAI's ChatGPT API to generate a response based on the input message. Here are the steps performed:
- It calls the openai.ChatCompletion.create() method, which sends a request to the API for generating a response based on a conversation. The create() method requires specifying the model to be used, in this case, "get-3.5-turbo", which is the GPT-3.5 model optimized for speed and efficiency.
2. The messages parameter is provided as a list of dictionaries. Each dictionary represents a message in the conversation and has two keys: "role" and "content". The "role" specifies the role of the message sender, which is set to "user" in this case. The "content" contains the actual text content of the message, which is passed as the msg parameter.
3. The create() method returns a response object, completion, which contains the generated completion or reply from the model.
4. The code converts the completion object to JSON format using json.dumps(), and then immediately parses it back to a Python dictionary using json.loads(). This conversion is done to extract the relevant information from the response.
5. Finally, the code accesses the content of the model's reply by retrieving the value of 'content' from the parsed JSON object. This reply is returned as the output of the chatgpt35() function.
THE SERVER SCRIPT
'''
File: smsgpt.py
Purpose: Serving an endpoint /sms that is receiving sms body and return json response to android phone via androidhelper
For: SMSGPT
Author: Mohamed
License: GNU General Public License version 2.0 or Later version
'''
from flask import Flask, request, jsonify
from gpt35 import chatgpt35
import json
import logging
logger = logging.getLogger(__name__)
logger.setLevel(logging.DEBUG)
ch = logging.StreamHandler()
ch.setLevel(logging.DEBUG)
formatter = logging.Formatter('%(asctime)s - %(name)s - %(levelname)s - %(message)s')
ch.setFormatter(formatter)
logger.addHandler(ch)
app = Flask(__name__)
@app.route("/")
def hello_world():
return jsonify({'SERVER_STATUS': 'OK'})
@app.route("/sms", methods=['POST'])
def process_sms():
data = request.get_json()
message = data['message']
logger.info(message)
return str(chatgpt35(message))
if __name__ == '__main__':
app.run(debug=True, host='0.0.0.0', port='8080')
The code above is defining a route for an endpoint in a Flask application. Let's break it down:
- @app.route("/sms", methods=['POST']) is a decorator that specifies the URL route ("/sms") and the HTTP methods (in this case, only "POST") that can be used to access this route. It associates the route with the following function, process_sms().
- def process_sms(): defines the function process_sms() that will be executed when a request is made to the specified route.
- data = request.get_json() retrieves the JSON payload from the request. It assumes that the request contains JSON data and uses the get_json() method from the request object to parse and extract the data.
- message = data['message'] extracts the value associated with the key 'message' from the JSON data. It assumes that the JSON data has a key named 'message' and assigns its value to the message variable.
- logger.info(message) logs the value of message using the info() method of a logger object. The specific logger object used is not shown in the provided code snippet.
- return str(chatgpt35(message)) calls the chatgpt35() function (that is defined in gpt35.py) with the message variable as an argument. The response from chatgpt35() is then converted to a string using str(). This string response is returned as the response to the request made to the "/sms" route.
In a nutshell, the above code defines a Flask route that expects a POST request with JSON data containing a key called 'message'. It retrieves the value of the 'message' key, logs it, and passes it as an argument to the chatgpt35() function. The response from chatgpt35() is then returned as a string response.
THE PHONE SIDE
Now on your phone with SMS Permissions allowed to QPython 3L create a file and paste the following code below, QPython will be running as daemon in the background allowing our script to be active all the time.
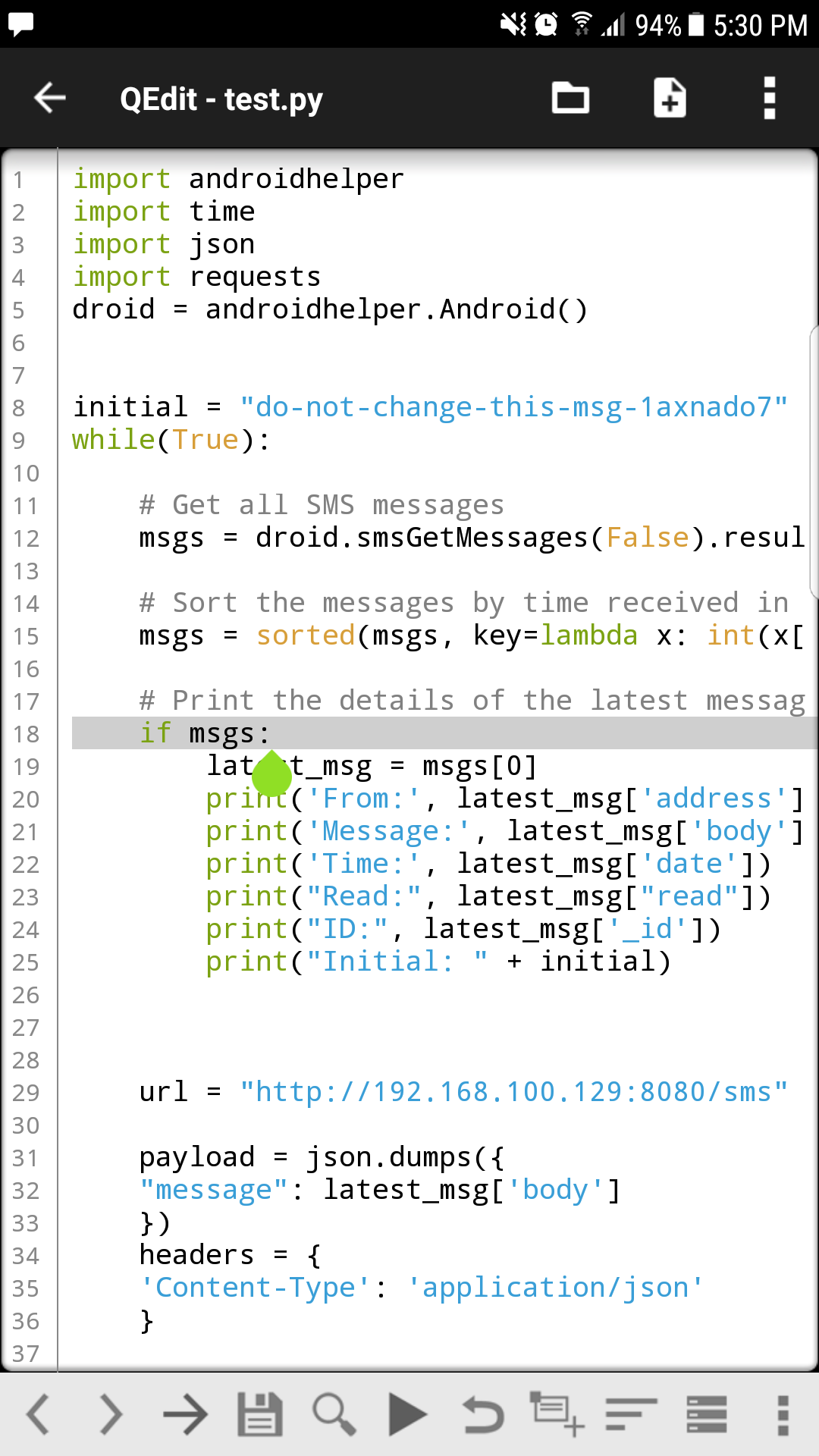
import androidhelper
import time
import json
import requests
droid = androidhelper.Android()
initial = "do-not-change-this-msg-for-starters"
while(True):
# Get all SMS messages
msgs = droid.smsGetMessages(False).result
# Sort the messages by time received in descending order
msgs = sorted(msgs, key=lambda x: int(x['date']), reverse=True)
# Print the details of the latest message received
if msgs:
latest_msg = msgs[0]
print('From:', latest_msg['address'])
print('Message:', latest_msg['body'])
print('Time:', latest_msg['date'])
print("Read:", latest_msg["read"])
print("ID:", latest_msg['_id'])
print("Initial: " + initial)
url = "http://192.168.100.129:8080/sms"
payload = json.dumps({
"message": latest_msg['body']
})
headers = {
'Content-Type': 'application/json'
}
if str(latest_msg['body']) != initial:
response = requests.request("POST", url, headers=headers, data=payload)
message = str(response.text)
if len(message) > 159:
chunk_size = 159
chunks = [message[i:i+chunk_size] for i in range(0, len(message), chunk_size)]
for i in range(len(chunks)):
#print(chunks[i])
droid.smsSend(str(latest_msg['address']), str(chunks[i]))
time.sleep(5)
print("Reponse form GPT: " + str(response.text))
initial = str(latest_msg['body'])
elif len(message) < 159:
droid.smsSend(str(latest_msg['address']), str(response.text))
print("Reponse form GPT: " + str(response.text))
initial = str(latest_msg['body'])
time.sleep(1)
The above code represents a loop that continuously checks for new SMS messages on an Android device, sends the latest received message to a specified URL, and sends the response from that URL back to the sender in the form of SMS messages. Here's a breakdown of the code:
initial = "do-not-change-this-msg-for-starters"
while True:
# Get all SMS messages
msgs = droid.smsGetMessages(False).result
# Sort the messages by time received in descending order
msgs = sorted(msgs, key=lambda x: int(x['date']), reverse=True)
# Print the details of the latest message received
if msgs:
latest_msg = msgs[0]
print('From:', latest_msg['address'])
print('Message:', latest_msg['body'])
print('Time:', latest_msg['date'])
print("Read:", latest_msg["read"])
print("ID:", latest_msg['_id'])
print("Initial: " + initial)
- The variable initial is assigned a string value of "do-not-change-this-msg-for-starters".
- The code enters an infinite loop using while(True):.
- It retrieves all SMS messages using droid.smsGetMessages(False).result and assigns the result to the variable msgs.
- The retrieved messages are sorted by the 'date' key in descending order using the sorted() function and a lambda function as the key parameter.
- If there are any messages (if msgs:), the details of the latest message are extracted from msgs[0] and printed, including the sender's phone number, message body, date, read status, and ID. The initial variable is also printed.
url = "http://192.168.100.129:8080/sms"
payload = json.dumps({
"message": latest_msg['body']
})
headers = {
'Content-Type': 'application/json'
}
6. A URL is defined as http://192.168.100.129:8080/sms. (My Local IP where the Flask server is running.)
7. The message body from the latest received SMS message is stored in the payload as a JSON object with a single key-value pair.
8. The headers for the HTTP request are set with 'Content-Type': 'application/json'.
if str(latest_msg['body']) != initial:
response = requests.request("POST", url, headers=headers, data=payload)
message = str(response.text)
if len(message) > 159:
chunk_size = 159
chunks = [message[i:i+chunk_size] for i in range(0, len(message), chunk_size)]
for i in range(len(chunks)):
droid.smsSend(str(latest_msg['address']), str(chunks[i]))
time.sleep(5)
print("Response from GPT: " + str(response.text))
initial = str(latest_msg['body'])
elif len(message) < 159:
droid.smsSend(str(latest_msg['address']), str(response.text))
print("Response from GPT: " + str(response.text))
initial = str(latest_msg['body'])
time.sleep(1)
9. The code checks if the body of the latest received message (latest_msg['body']) is different from the initial message. If they are not the same, the following actions are taken:
10. A POST request is made to the specified URL using requests.request() with the provided URL, headers, and payload data.
11. The response from the URL is converted to a string and stored in a message.
12. If the length of the response (message) is greater than 159 characters, it is split into smaller chunks of 159 characters using a list comprehension. Each chunk is stored in the chunks list.
13. For each chunk in the chunks list, the Android device sends an SMS to the sender's phone number (stored in latest_msg['address']) containing the chunk of the response message. The droid.smsSend() method is used for sending SMS messages. After sending each chunk, the code pauses for 5 seconds using time.sleep(5).
14. The response from the URL (the full response message) is printed using print("Response from GPT: " + str(response.text)).
15. The initial variable is updated to the body of the latest received message (initial = str(latest_msg['body'])).
16. If the length of the response message (message) is less than 159 characters, the Android device sends an SMS containing the full response message to the sender's phone number. The response message is printed, and the initial variable is updated.
17. After sending the SMS response, the code pauses for 1 second using time.sleep(1).
18. The code continuously loops through these steps, checking for new SMS messages, sending them to the specified URL, and sending the response back via SMS.
SUMMARY AND CONCLUSION
The provided information introduces the concept of integrating ChatGPT, an artificial intelligence model, with SMS messaging services using Python. This integration aims to leverage the power of natural language processing and machine learning to create dynamic and intelligent conversations through SMS. By utilizing the Androidhelper library, users can employ their Android phones as SMS gateway.
To implement the integration, certain requirements must be met, including having Python installed, access to ChatGPT, and installing necessary Python libraries such as requests, Flask, and OpenAI. Additionally, on the Android phone, the QPython3L and Androidhelper libraries need to be installed, and the phone should be configured as SMS Gateway.
The provided code includes a Python script, 'gpt35.py', which serves as a wrapper for making API calls to the ChatGPT model. It utilizes OpenAI's ChatCompletion API to generate responses based on user messages. The code defines a function called 'chatgpt35', which takes a message as input and returns the generated response from the ChatGPT model.
Another script, 'smsgpt.py', sets up a Flask application with two routes. The "/sms" route is configured to receive POST requests with JSON payloads containing SMS messages. It extracts the message from the request, passes it to the 'chatgpt35' function, and returns the response as a string.
The final script, 'test.py', runs on the Android phone as a daemon process. It continuously checks for new SMS messages, sends the latest message to the Flask server's "/sms" route as a JSON payload, and retrieves the response. If the response exceeds 159 characters, it splits it into smaller chunks and sends them as separate SMS messages. Otherwise, it sends the full response as an SMS.
In summary, the integration of ChatGPT with SMS services allows for intelligent and dynamic conversations through text messages. By following the provided steps and using the provided code snippets, users can bring the power of artificial intelligence to their SMS communication. The combination of ChatGPT and SMS opens up possibilities for personalized responses, improved engagement, and time-saving interactions, revolutionizing the way people communicate through text messages.
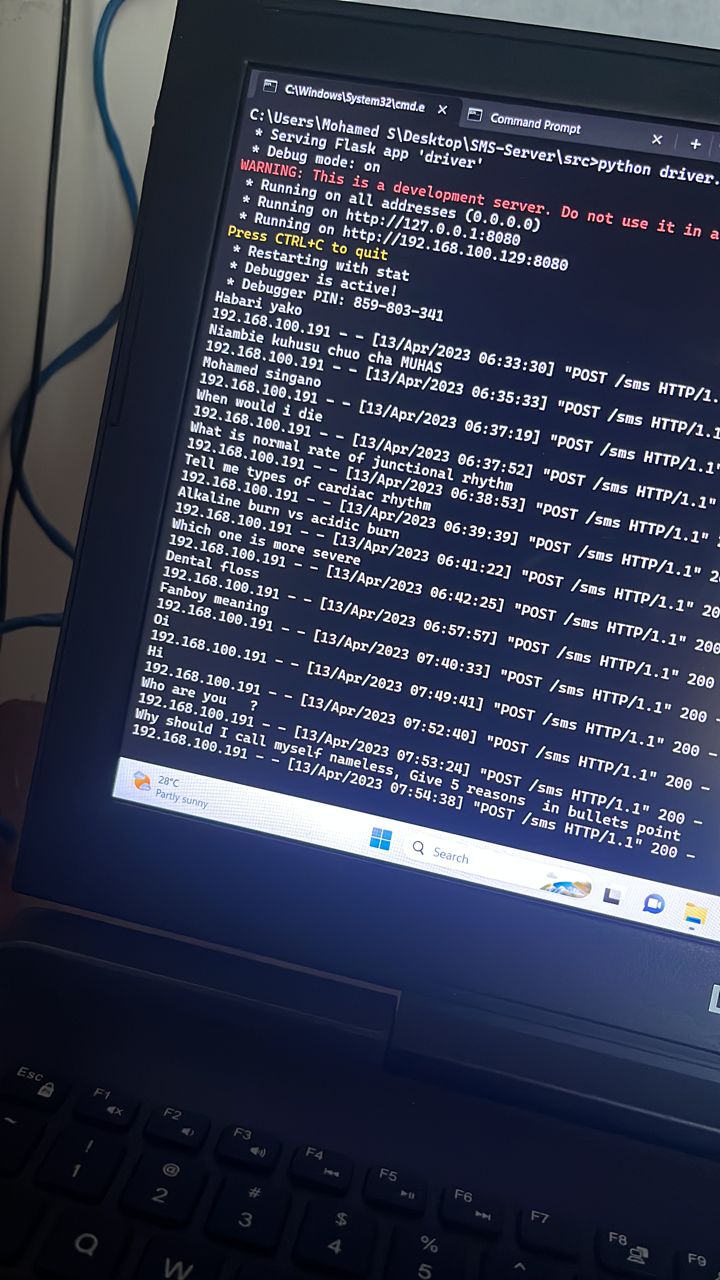
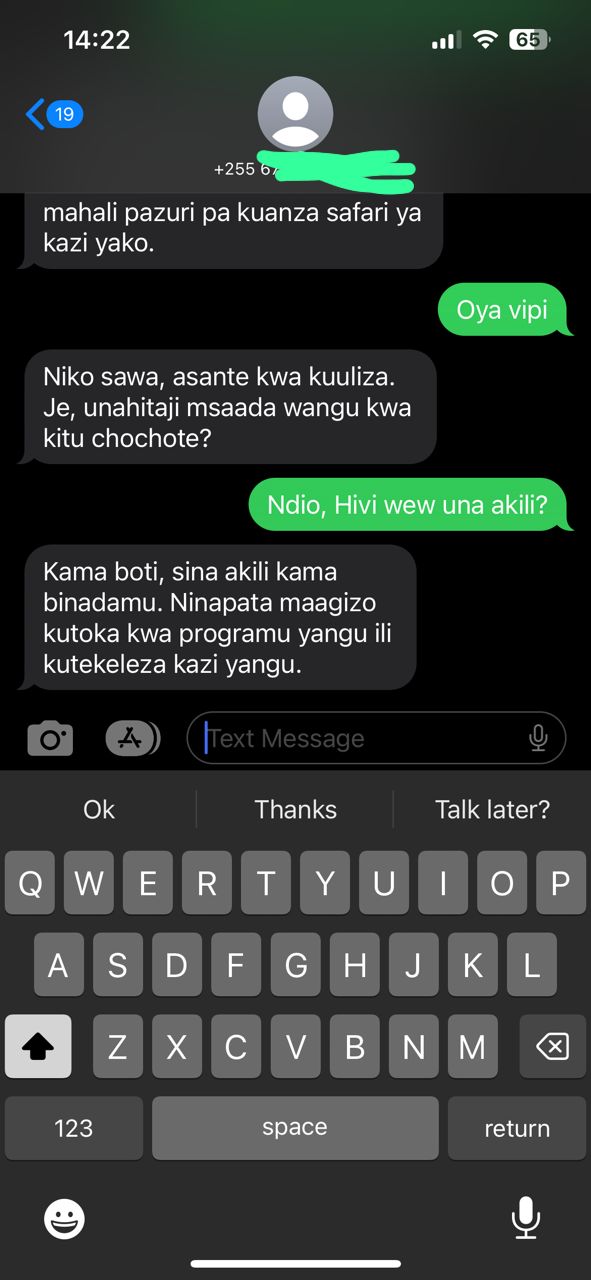